Quick Look at GoogleTest for C++
A quick look at GoogleTest library for automated testing in C++.
Installation
First, you need to install GoogleTest, which can be done by following the instructions at https://github.com/google/googletest.
Example
This example will be a simplified, and poorly written, calculator for signed-integers (a-ha!), “suitably” named calc
, and
it supports addition and subtraction.
The header file calc.hpp
:
The implementation file calc.cpp
:
And the test file calc_test.cpp
:
There is some stuff from GoogleTest:
- Its header file
gtest/gtest.h
. - The
TEST
macro creates the test cases, where the first parameter is the test-suite name and the second is the test-case name. - The
ASSERT_EQ
, member of theASSERT_*
group of macros, which asserts for equality, stopping execution if the arguments are not equal. You may also useEXPECT_EQ
if you want to continue execution regardless. - You can set GoogleTest up by calling
testing::InitGoogleTest
. - Then, start GoogleTest up by invoking
RUN_ALL_TESTS
.
Compilation
Depending on your setup and how you’ve installed the library, you may be able to compile by typing:
g++ -o calc_test calc_test.cpp calc.cpp -lgtest -lpthread
Note that you must link the code against the GoogleTest ( gtest ) and the POSIX Thread ( pthread ) libs.
Running
To run the test suite, just type:
./calc_test
Thus, the test runner will run each test-suite and print a summary similar to this:
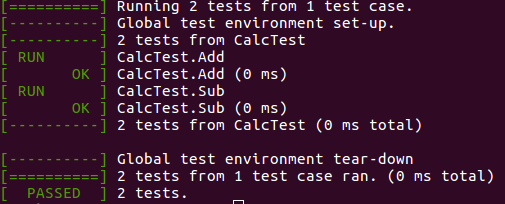
Conclusion
During software development, life-cycle is important to write tests to check for correctness, thereby paving the way for improvements later down the road by boosting developer’s confidence when refactoring, etc.
In this context, tools for automated testing are critical to helping us out in the process. They should be integrated into Continuous Integration (CI) pipelines to be executed automatically upon pushes.
References
[1] https://github.com/google/googletest#Assertions
[2] https://www.eriksmistad.no/getting-started-with-google-test-on-ubuntu/
Originally published at https://medium.com/@rvarago